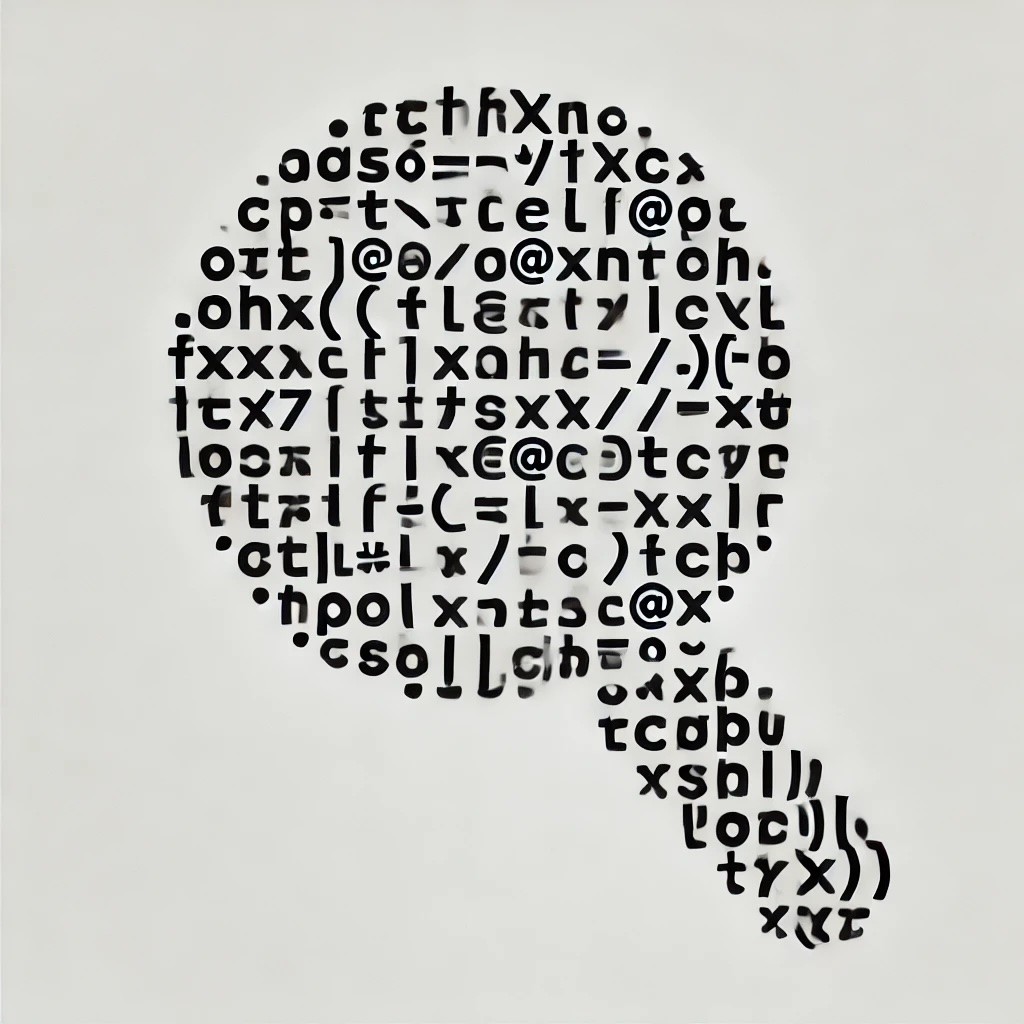
MiniGrep
Project Overview
A command-line search tool implemented in Rust that demonstrates core systems programming concepts, developed as part of The Rust Programming Language book. The project showcases Rust's safety features, error handling patterns, and file I/O capabilities while providing a practical utility for text searching.
Key Features
Core Functionality
- Case-Sensitive Search: Configurable case sensitivity through environment variables
- File Processing: Efficient file reading and content searching
- Error Handling: Robust error management using Rust's Result type
- Environment Configuration: Runtime behavior modification through environment variables
Implementation Details
Search Implementation
pub fn search<'a>(query: &str, contents: &'a str) -> Vec<&'a str> {
contents
.lines()
.filter(|line| line.contains(query))
.collect()
}
pub fn search_case_insensitive<'a>(
query: &str,
contents: &'a str,
) -> Vec<&'a str> {
let query = query.to_lowercase();
contents
.lines()
.filter(|line| line.to_lowercase().contains(&query))
.collect()
}
Configuration Management
pub struct Config {
pub query: String,
pub file_path: String,
pub ignore_case: bool,
}
impl Config {
pub fn build(
mut args: impl Iterator<Item = String>,
) -> Result<Config, &'static str> {
args.next();
let query = match args.next() {
Some(arg) => arg,
None => return Err("Didn't get a query string"),
};
let file_path = match args.next() {
Some(arg) => arg,
None => return Err("Didn't get a file path"),
};
let ignore_case = env::var("IGNORE_CASE").is_ok();
Ok(Config {
query,
file_path,
ignore_case,
})
}
}
Technical Capabilities
Error Handling
Comprehensive error handling using Rust's Result type and error propagation
Memory Safety
Zero-cost abstractions with Rust's ownership and borrowing system
Configuration
Environment-based configuration with flexible runtime behavior
Learning Outcomes
Key Concepts Demonstrated
- Rust ownership and borrowing patterns
- Error handling best practices
- Command-line argument parsing
- Environment variable usage
- File I/O operations
- Test-driven development